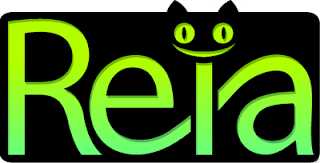
Reia es un lenguaje de programación script inspirado en Ruby que corre sobre la maquina virtual de Erlang. Reia une lo mejor de los dos mundos Ruby y Erlang. Reia tiene reflexion, meta-programación, bloques de código, manejo de concurrencia mediante el modelo de actores, distribuido, hot code y tolerancia a fallos.
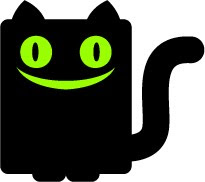
# Hello, world!
"Hello, world!".puts()
# Assignment
number = 42
opposite = true
# Conditions
number = -42 if opposite
# Lists (stored as immutable singly-linked lists)
list = [1, 2, 3, 4, 5]
# Tuples (think of them as immutable arrays)
tuple = (1, 2, 3, 4, 5)
# Atoms (known as symbols to Ruby people)
# Think of them as an open-ended enumeration
atom = :up_and_atom
# Dicts (also known as hashes to Ruby people)
dict = {:foo => 1, :bar => 2, :baz => 3}
# Strings (unlike Erlang, Reia has a real String type!)
string = "I'm a string! Woohoo I'm a string! #{'And I interpolate too!'}"
# Ranges
range = 0..42
# Funs (anonymous functions, a.k.a. lambdas, procs, closures, etc.)
# Calling me with plustwo(40) would return 42
plustwo = fun(n) { n + 2 }
# Modules (collections of functions)
# Calling Plusser.addtwo(40) would return 42
module Plusser
def addtwo(n)
n + 2
end
end
# Classes (of immutable objects. Once created objects can't be changed!)
class Adder
# Reia supports binding instance variables directly when they're passed
# as arguments to initialize
def initialize(@n); end
def plus(n)
@n + n
end
end
# Instantiate classes by calling Classname(arg1, arg2, ...)
# For you Ruby people who want Classname.new(...) this is coming soon!
fortytwo = Adder(40).plus(2)
# Function references can be obtained by omitting parens from a function call,
# like JavaScript or Python
numbers = [1,2,3]
reverser = [1,2,3].reverse
# Function references can be invoked just like lambdas
reversed = reverser() # reversed is now [3,2,1]
# You can add a ! to the end of any method to rebind the method receiver to
# the return value of the given method minus the bang.
numbers.reverse!() # numbers is now [3,2,1]
# List comprehensions
doubled = [n * 2 for n in numbers] # doubled is [6,4,2]
### Numbers
42 # integer
4.2 # float
### Booleans
true # All expressions evaluate true in a boolean context except false and nil
false
nil
### Atoms (an open enumeration, like Ruby's symbols)
:foobar # A simple atom
:"I am the very model of a modern major general" # quoted
### Lists
list = [3,4,5] # lists are singly linked and good for ordered sequences
list2 = [1,2,*list] # the splat operator lets you prepend to an existing list
[1,2,3,*rest] = list2 # splats match patterns. rest is now [4,5]
five = list[2] # List#[] retrieves the nth (zero indexed) member
### Tuples
tuple = (1,2,3) # tuples are immutable arrays
two = tuple[1] # Tuple#[] retrieves the nth (zero indexed) member
# Tuple#[]= produces new versions of a tuple and binds them to the receiver
tuple[1] = 42 # tuple is now (1,42,3)
tuple[2] += 40 # tuple is now (1,42,43)
### Dicts
dict = {:foo => 1, :bar => 2, :baz => 3} # Dicts are key/value maps
bar = dict[:bar] # Use Dict#[] to retrieve a value for a given key. bar is 2
dict[:bar] += 40 # Dict#[]= alters the value of a member
### Strings
"Ohai!" # strings can be double quoted
'Ohai!' # or single quoted
"Ohai, #{name}!" # double quoted strings interpolate
'Ohai, #{name}!' # single quoted strings interpolate too
### Binaries
<[1,2,3]> # binaries allow you to store binary data without stringy fluff
<["string"]> # you can make binaries from strings, too
<[1,2,x]> = <[1,2,3]> # pattern matching can be used with binaries too
### Regexps
%r/^.{3}/ # I'm a regex literal! (Soon %r will go away and /.../ will work)
%r/^.{3}/.match("foo bar baz") # Returns "foo"
### Ranges
1..10 # I'm a range!
(1..10).to_list() # Returns [1,2,3,4,5,6,7,8,9,10]
[n * 2 for n in 1..10, n % 2 == 1] # List comprehension of a range, returns [2,6,10,14,18]
### Funs (a.k.a. anonymous functions or lambda expressions)
adder = fun(x, y) { x + y }
adder(40, 2) # returns 42
multipler = fun(x, y) do
x * y
end
multiplier(7, 6) # returns 42
Como siempre dejo link: